RKNN | Company Info
JavaScript Interview Questions about SPA State Data Structures for 2020
If you are still asking about the for-loop syntax or want the candidates to list the JavaScript data types in your web development interviews, this might be for you.
We are doing a lot of Single Page Application development using one of the big three frameworks (Angular, React, Vue.js) in our daily work as web and JavaScript developers. The experience we habe been collecting for a lot of years now combined with our experience from all the projects we did provided us with a much clearer picture on what Single Page App development mainly means nowadays.
Skills for JavaScript SPA development in 2020
From our point of view, developing a Single Page Application nowadays is mainly about app architecture, state management and data flow throughout the web application. So one of the key tasks is to transfer the business requirements into the web applications data structure whilst still respecting state management best practices.
Still asking about for-loop syntax?
We have put together a very basic data structure task we might ask for as part of an interview.
We'd like to demonstrate the idea, as well as share some sample interview questions. This is of course intended to just scratch the surface of data structure transformation in JavaScript or TypeScript. We are very looking forward to every feedback and to hear about your experiences when hiring or getting hired in this field. No matter if you agree or disagree with us, let us know.
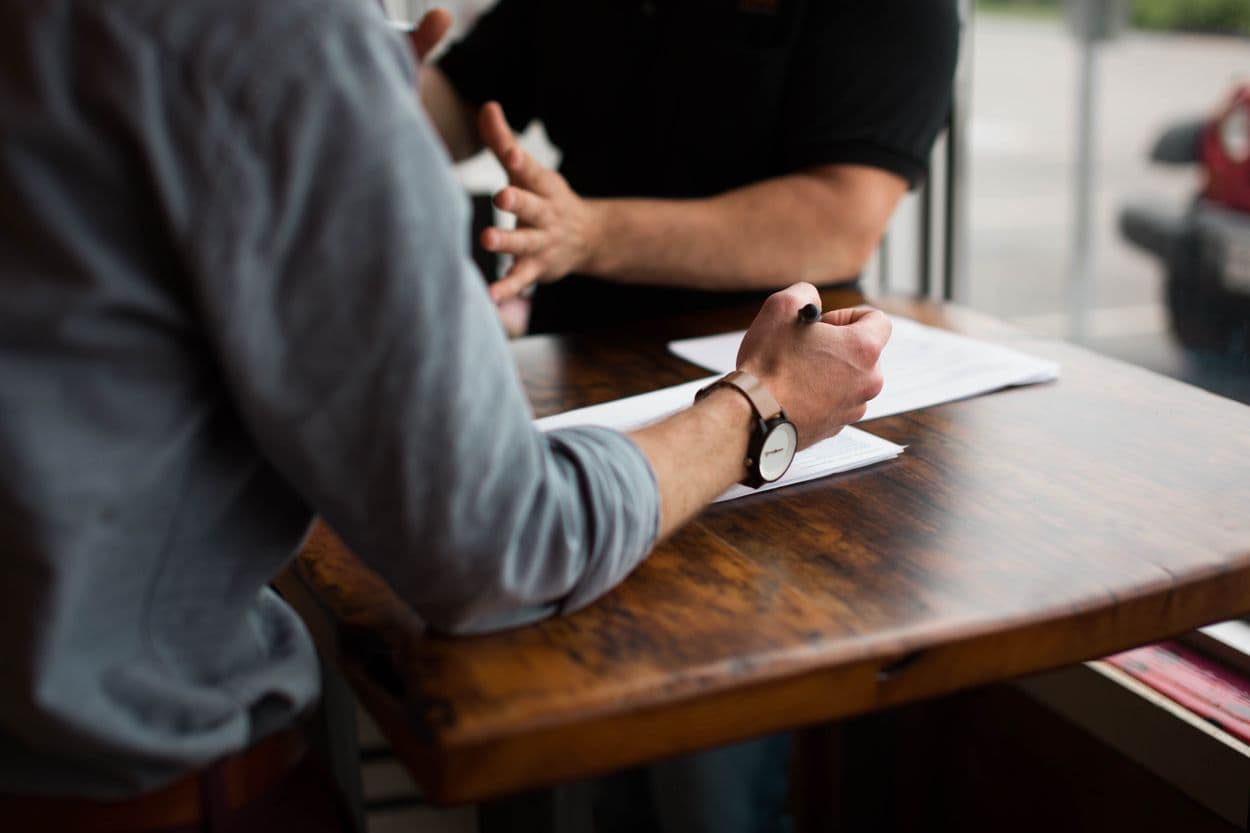
But which framework are the questions for?
But where is the framework-specific syntax you are asking yourself? We consider this valid no matter which JavaScript SPA Framework and State Management library/approach we are hiring for. Those questions are relevant for how data is transformed in JavaScript. For us as a web application development company which is not focused on one framework, we don't care too much about the peculiarities of any single framework but tend to focus on the general JavaScript skills our developers must possess. We prioritize mastering the underlying patterns and high-level concepts. And handling data structures is one of them. So for us, this knowledge belongs to the things every serious SPA developer will need to address sooner or later. Let's start.
Example #1
Let's assume you get the following data from an API Response:
const apiResponse = [
{id: 'id1', name:'one'},
{id: 'id2', name:'two'}
]
You want to store this data in the app state. Therefore you want to change it a bit for better state management. You want to transform the data into the following structure:
{
id1: {id: 'id1', name:'one'},
id2: {id: 'id2', name:'two'}
}
Your task: Please create the code to restructure the shown apiResponse
into the target data structure.
Example #2
Assume you are getting this data structure from your app state and want to display it in a component:
const appStateData = {
id1: {id: 'id1', name:'one'},
id2: {id: 'id2', name:'two'}
}
Your Task: Please modify the given data into the following data structure to be used in the components id based iterator:
{
ids: ['id1', 'id2'],
names: ['one', 'two']
}
Example #3
Assume you have a data structure that looks like this in your current app state:
const currentState = {
byId: {
id1: {id: 'id1', name:'one'},
id2: {id: 'id2', name:'two'}
},
byName: {
one: {id: 'id1', name:'one'},
two: {id: 'id2', name:'two'}
},
}
Imagine an API provides you with a new piece of data that looks like this:
const apiResponse = [
{id: 'id3', name:'three'}
]
Your task here is to write the code that merges the apiResponse
into the currentState
, which should result in the following data structure
{
byId: {
id1: {id: 'id1', name:'one'},
id2: {id: 'id2', name:'two'},
id3: {id: 'id3', name:'three'}
},
byName: {
one: {id: 'id1', name:'one'},
two: {id: 'id2', name:'two'},
three: {id: 'id3', name:'three'}
},
}
Solutions
Of course there is more than one solution to every example. And of course there might not be one solution that is in general 'the best' one. It all depends on the context. But let's at least see how it might be done.
Solution to Example #1
We would be happy to see a reduce here, for example:
apiResponse.reduce((acc, row)=>{
acc[row.id] = row;
return acc;
}, {});
Or even shorter:
apiResponse.reduce((acc, row)=> ({...acc, [row.id]: row}), {});
Solution to Example #2
We would be happy to see a reduce here, for example:
Object.values(appStateData).reduce((acc, row)=>{
acc.ids.push(row.id);
acc.names.push(row.name);
return acc;
}, {ids: [], names: []});
Or even shorter:
Object.values(appStateData).reduce((acc, row) => ({
ids: [...acc.ids, row.id],
names: [...acc.names, row.name]
}), { ids: [], names: [] });
Solution to Example #3
Usually we would prefer this as one statement, but for readability in this post we broke it down into three separate steps:
const newByIdEntries = apiResponse.reduce((acc, obj)=>({...acc, [obj.id]: obj}), {});
const newByNameEntries = apiResponse.reduce((acc, obj)=>({...acc, [obj.name]: obj}), {});
const newState = {
...currentState,
byId: { ...currentState.byId, ...newByIdEntries},
byName: { ...currentState.byName, ...newByNameEntries}
}
Candidates, you are welcome
If you are a candidate looking to apply or already preparing for an interview at RKNN: you are welcome for this little hint, but beware...this is by far not all you need to know to land a job here.

Mark Heyermann
Berater und Entwickler. Gründer und Geschäftsführer der RKNN GmbH.